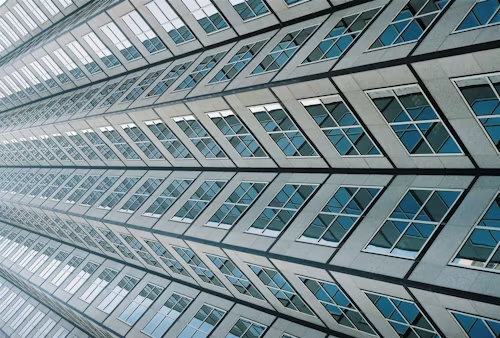
Introduction
In the world of software development, creating clean, maintainable, and scalable systems is a top priority. One way to achieve this is by using design patterns — time-tested solutions to recurring design problems. These patterns offer developers a shared language and structured approach to software design, ensuring consistency, flexibility, and ease of maintenance across projects. Whether you’re a beginner or an experienced developer, understanding design patterns can significantly improve your ability to solve complex problems efficiently.
In this article, we will explore the concept of design patterns, their types, and provide real-world examples. We will dive deep into how design patterns can elevate your development process by making it more structured, reusable, and scalable.
What Are Design Patterns?
At their core, design patterns are reusable solutions to common software design problems that developers face during the development process. They are blueprints that describe how to solve a particular problem or design challenge in a flexible and scalable way. While design patterns are not ready-to-use code, they offer conceptual solutions that developers can adapt to suit specific needs.
Imagine a seasoned developer with years of experience who knows the pitfalls of a particular design challenge. Instead of reinventing the wheel, they employ a pattern that has already been proven to work in similar situations. These patterns allow developers to create systems that are not only functional but also easy to modify, extend, and scale as business requirements evolve.
The concept of design patterns gained prominence in the early 1990s with the publication of Design Patterns: Elements of Reusable Object-Oriented Software by Erich Gamma, Richard Helm, Ralph Johnson, and John Vlissides (collectively known as the “Gang of Four”). This book outlined 23 classic design patterns and laid the foundation for modern software design.
Why Are Design Patterns Important?
The importance of design patterns in software engineering cannot be overstated. Here’s why:
- Code Reusability: By using design patterns, developers can reuse tried and tested solutions, reducing the need to create custom solutions from scratch.
- Maintainability: Patterns offer a consistent approach to coding that helps keep the system maintainable. They provide clarity in complex systems, making it easier for other developers to understand and extend the codebase.
- Flexibility: Many design patterns promote flexibility by decoupling components in the system. This decoupling allows developers to modify or replace parts of the system with minimal impact on the overall architecture.
- Efficiency: Design patterns often optimize both time and resources. Instead of solving a problem from the ground up, a developer can apply an existing solution, speeding up the development process.
In addition, using design patterns can lead to better software architecture, improving both the functionality and the robustness of a system.
Types of Design Patterns
Design patterns are typically categorized into three broad groups: Creational, Structural, and Behavioral. Each category addresses a different aspect of software design, from object creation to how objects interact and communicate with each other.
1. Creational Design Patterns
Creational design patterns are focused on object creation mechanisms. They abstract the instantiation process to make the system more flexible and independent of the object creation process. This category is particularly useful when the system needs to create objects in various configurations or requires flexibility in object creation.
Common Creational Patterns:
- Singleton Pattern: The Singleton pattern ensures that a class has only one instance and provides a global point of access to that instance. This pattern is particularly useful for managing shared resources such as database connections or configuration settings.For example, a database connection pool that should only have one instance throughout the application’s lifetime would be a good candidate for the Singleton pattern.
class Singleton:
_instance = None
def __new__(cls):
if cls._instance is None:
cls._instance = super(Singleton, cls).__new__(cls)
return cls._instance
- Factory Method Pattern: The Factory Method pattern defines an interface for creating objects but allows subclasses to alter the type of objects that will be created. This pattern is helpful when the exact type of the object to be created is determined at runtime.For example, in a graphical user interface (GUI) system, different button types (e.g., Windows, macOS, Linux) can be created based on the operating system without changing the client code.
class Shape:
def draw(self):
pass
class Circle(Shape):
def draw(self):
return "Drawing a Circle"
class ShapeFactory:
def create_shape(self, shape_type):
if shape_type == 'circle':
return Circle()
else:
raise ValueError("Unknown shape type")
2. Structural Design Patterns
Structural design patterns deal with how objects and classes are composed to form larger structures. These patterns ensure that the system remains efficient, organized, and easy to extend.
Common Structural Patterns:
- Adapter Pattern: The Adapter pattern allows objects with incompatible interfaces to work together. It acts as a bridge between two incompatible systems, allowing them to communicate without changing their code.For example, if you have a legacy system that reads data from one format and a modern system that requires a different format, you could use an adapter to bridge the gap.
class LegacySystem:
def old_method(self):
return "Data from old system"
class Adapter:
def __init__(self, legacy_system):
self.legacy_system = legacy_system
def new_method(self):
return self.legacy_system.old_method()
- Composite Pattern: The Composite pattern lets you treat individual objects and compositions of objects uniformly. This is ideal for representing part-whole hierarchies, such as a file system where both files and directories are treated similarly.
class Component:
def operation(self):
pass
class Leaf(Component):
def operation(self):
return "Leaf operation"
class Composite(Component):
def __init__(self):
self.children = []
def add(self, component):
self.children.append(component)
def operation(self):
return "Composite operation with: " + ", ".join(child.operation() for child in self.children)
3. Behavioral Design Patterns
Behavioral design patterns focus on the interaction between objects and the delegation of responsibilities. These patterns make it easier to manage how objects communicate, collaborate, and share responsibilities.
Common Behavioral Patterns:
- Observer Pattern: The Observer pattern defines a one-to-many relationship between objects, such that when one object changes state, all its dependents are notified and updated automatically.For example, a weather station may notify various display systems when there is a change in weather data (e.g., temperature, humidity).
class Observer:
def update(self, temperature):
pass
class WeatherStation:
def __init__(self):
self._observers = []
def add_observer(self, observer):
self._observers.append(observer)
def notify_observers(self, temperature):
for observer in self._observers:
observer.update(temperature)
class TemperatureDisplay(Observer):
def update(self, temperature):
print(f"Temperature updated to: {temperature}")
- Strategy Pattern: The Strategy pattern defines a family of algorithms, encapsulates each one, and makes them interchangeable. The client can choose which algorithm to use at runtime, making this pattern ideal for situations where multiple strategies can be applied to solve a problem.For example, a payment system might support multiple payment methods (e.g., Credit Card, PayPal, Bitcoin), and the user can select which one to use.
class PaymentStrategy:
def pay(self, amount):
pass
class CreditCardPayment(PaymentStrategy):
def pay(self, amount):
print(f"Paid {amount} using Credit Card")
class PayPalPayment(PaymentStrategy):
def pay(self, amount):
print(f"Paid {amount} using PayPal")
class PaymentContext:
def __init__(self, strategy: PaymentStrategy):
self.strategy = strategy
def execute_payment(self, amount):
self.strategy.pay(amount)
Conclusion
Design patterns are an essential part of software engineering, offering tried-and-true solutions to common design challenges. By incorporating design patterns into your projects, you can improve code reusability, maintainability, scalability, and flexibility. These patterns help developers avoid common pitfalls and provide a structured way to tackle complex problems. Whether you’re using creational, structural, or behavioral patterns, each pattern serves a unique purpose and can be applied across different scenarios to make your code more efficient and maintainable.
As you continue to grow as a software developer, mastering design patterns will empower you to create more robust and scalable applications. By applying the right design pattern to the right problem, you can write cleaner, more efficient, and more flexible code.